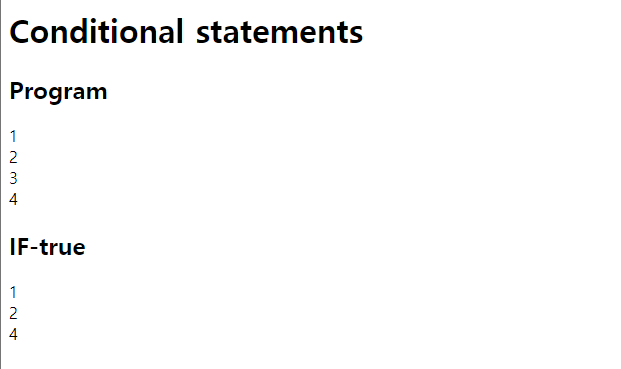
16장. 조건문
ex5.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
</head>
<body>
<h1>Conditional statements</h1>
<script>
</script>
</body>
</html>
프로그램을 짜 본다.
<body>
<h1>Conditional statements</h1>
<script>
document.write("1<br>");
document.write("2<br>");
document.write("3<br>");
document.write("4<br>");
</script>
</body>
아래에 다음과 같은 코드를 추가한다.
<h2>IF-true</h2>
<script>
document.write("1<br>");
if(true) {
document.write("2<br>");
}
else {
document.write("3<br>");
}
document.write("4<br>");
</script>
아래 프로그램에서는 true가 아닐 때, 3이 제외되고 출력된 것을 확인할 수 있다.
<h2>IF-true</h2>
<script>
document.write("1<br>");
if(false) {
document.write("2<br>");
}
else {
document.write("3<br>");
}
document.write("4<br>");
</script>
위와 같이 코드를 수정하면, 아래처럼 2만 제외하고 결과가 나타난다.
불리언 (true, false)에 따라 결과가 달라지는 것이 조건문의 핵심이다.
17장. 조건문의 활용
앞선 예제의 index.html에서 night, day 버튼을 하나로 만들어보자.
<input type="button" value="night" onclick="">
class="but"으로 설정한다.
<input type="button" value="night" class="but" onclick="">
night 버튼의 value 값이 night라면 night 부분의 코드가, night가 아니라면 day 부분의 코드가 실행되도록 기능을 구현해 보자. 이를 위해서 현재 버튼의 value 값을 알아내야 한다, 그 코드는 아래와 같다.
document.querySelector('#night_day').value
id가 night_day인 버튼의 value를 가져오는 코드이다.
이를 적용해 아래와 같이 코드를 작성할 수 있다.
<input id="night_day" type="button" value="night" class="but" onclick="
if (document.querySelector('#night_day').value === 'night') {
document.querySelector('body').style.backgroundColor = 'black';
document.querySelector('body').style.color = 'white';
document.querySelector('#night_day').value = 'day';
}
else {
document.querySelector('body').style.backgroundColor = 'white';
document.querySelector('body').style.color = 'black';
document.querySelector('#night_day').value = 'night';
}
">
이 버튼 하나만으로 야간, 주간 모드를 모두 적용할 수 있다.
18장. 중복의 제거를 위한 리팩터링
refactoring : 코드의 비효율적인 면을 제거하고 효율적으로 만들어 코드의 가독성을 높이고, 유지보수를 편리하게 만들며, 중복된 코드를 줄이는 방향으로 코드를 개선하는 작업
<input type="button" value="night" class="but" onclick="
if (this.value === 'night') {
document.querySelector('body').style.backgroundColor = 'black';
document.querySelector('body').style.color = 'white';
this.value = 'day';
}
else {
document.querySelector('body').style.backgroundColor = 'white';
document.querySelector('body').style.color = 'black';
this.value = 'night';
}
">
this는 바로 이 이벤트를 가리키는 것이므로 중복을 제거할 수 있다.
<input type="button" value="night" class="but" onclick="
var target = document.querySelector('body')
if (this.value === 'night') {
target.style.backgroundColor = 'black';
target.style.color = 'white';
this.value = 'day';
}
else {
target.style.backgroundColor = 'white';
target.style.color = 'black';
this.value = 'night';
}
">
또한 target이라는 변수를 지정해 document.querySelector('body')를 저장하여 중복된 부분을 제거하였다.
19장. 반복문 예고
"반복문" 을 이용하면, 코드의 양을 획기적으로 줄일 수 있다.
20장. 배열
데이터 중에서 서로 연관된 데이터를 잘 정리 정돈해서 담아두는, 일종의 수납 상자
칸은 여러 개.
var coworkers = ["egoing", "leezche"];
데이터 타입에 cowerkers라는 이름을 붙인 것이다. (변수 사용)
ex6.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
</head>
<body>
<h1>Array</h1>
<h2>Syntax</h2>
<script>
var coworkers = ["egoing", "leezche"];
</script>
<h2>get</h2>
<script>
document.write(coworkers[0]);
document.write(coworkers[1]);
</script>
</body>
</html>
coworker 배열에 변수 2개를 저장하고 이를 document에 표시해 보았다, 출력 결과
배열의 길이는 아래와 같은 식으로 확인할 수 있다.
document.write(coworkers.length);
베열의 길이인 2가 표시된다.
데이터를 추가하고 싶을 때는 push를 사용한다.
<script>
document.write(coworkers[0]);
document.write(coworkers[1]);
document.write(coworkers.length);
</script>
<h2>add</h2>
<script>
coworkers.push("duru");
coworkers.push("taeho");
</script>
<h2>count</h2>
<script>
document.write(coworkers.length);
</script>
배열의 길이가 4로 잘 늘어난 것을 확인할 수 있다.
21장. 반복문
반복문 (=루프)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>loop</h1>
<ul>
<script>
document.write('<li>1</li>');
document.write('<li>2</li>');
document.write('<li>3</li>');
document.write('<li>4</li>');
document.write('<li>5</li>');
</script>
</ul>
</body>
</html>
조금 변형해보자.
<body>
<h1>loop</h1>
<ul>
<script>
document.write('<li>1</li>');
var i = 0;
while(i < 3) {
document.write('<li>2</li>');
document.write('<li>3</li>');
i++;
}
document.write('<li>5</li>');
</script>
</ul>
</body>
실행 결과 반복문의 삽입으로 인해 2 3이 3회 반복된다.
22장. 배열과 반복문
ex8.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Loop & Array</h1>
<h2>Co workers</h2>
<ul>
<li>egoing</li>
<li>leezche</li>
<li>duru</li>
<li>taeho</li>
</ul>
</body>
</html>
반복문을 사용하면 훨씬 간단한 코드로 바꿀 수 있다.
<body>
<h1>Loop & Array</h1>
<script>
var coworkers = ['egoing', 'leezche', 'duru', 'taeho'];
</script>
<h2>Co workers</h2>
<ul>
<script>
var i = 0;
while (i < 4) {
document.write('<li>' + coworkers[i] + '</li>')
i++;
}
</script>
</ul>
</body>
아래와 같이 고칠 수도 있다.
<ul>
<script>
var i = 0;
while (i < coworkers.length) {
document.write('<li>' + coworkers[i] + '</li>')
i++;
}
</script>
</ul>
23장. 배열과 반복문의 활용
var aiist = document.querySelectorAll('a');
이는 alist라는 배열에 태그 a를 가진 요소들을 저장하는 것이다. 인덱스를 이용해 이를 차례로 꺼내볼 수 있다.
반복문을 이용한다.
<script>
var alist = document.querySelectorAll('a');
var i = 0;
while (i < alist.length) {
console.log(alist[i]);
i++;
}
</script>
해당하는 요소들이 순서대로 잘 출력되었다.
이제 요소의 색을 바꾸어 보자,
<script>
var alist = document.querySelectorAll('a');
var i = 0;
while (i < alist.length) {
console.log(alist[i]);
alist[i].style.color = 'powderblue';
i++;
}
</script>
색깔이 잘 바뀌었다. 이제 이를 버튼에 적용해보자.
var target = document.querySelector('body');
var alist = document.querySelectorAll('a');
if (this.value === 'night') {
target.style.backgroundColor = 'black';
target.style.color = 'white';
var i = 0;
while (i < alist.length) {
console.log(alist[i]);
alist[i].style.color = 'powderblue';
i++;
}
this.value = 'day';
}
else {
target.style.backgroundColor = 'white';
target.style.color = 'black';
var j = 0;
while (j < alist.length) {
console.log(alist[j]);
alist[j].style.color = 'blue';
j++;
}
this.value = 'night';
}
성공적으로 적용되었다.
24장. 함수 예고
함수 = 영어로 function, 수납상자
function nightDayHandler(self)
25장. 함수
ex9.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Function</h1>
<h2>Basic</h2>
<ul>
<script>
document.write('<li>1</li>');
document.write('<li>2-1</li>');
document.write('<li>2-2</li>');
document.write('<li>3</li>');
</script>
</ul>
</body>
</html>
동작을 반복해야 하는 경우이나 반복문을 사용할 수 없을 때, 함수를 사용해 이를 쉽게 나타낼 수 있다.
<ul>
<script>
function two(){
document.write('<li>2-1</li>');
document.write('<li>2-2</li>');
}
document.write('<li>1</li>');
two();
document.write('<li>3</li>');
two();
</script>
</ul>
'생활코딩! HTML+CSS+자바스크립트' 카테고리의 다른 글
WEB 5주차 (Javascript 7~15장) (0) | 2023.09.03 |
---|---|
WEB 4주차 (Javascript 1~6장) (0) | 2023.08.26 |
WEB 3주차 (CSS 7장~15장) (0) | 2023.08.20 |
WEB 2주차 (HTML 17~22장, CSS 1~6장) (0) | 2023.08.13 |
WEB 1주차 (HTML 1~16장) (0) | 2023.08.05 |